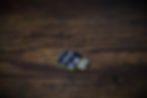
Anytime you get new tech to play with one of the first things to do is play around. Goofing off in a sense is a great way to learn something fast. When I got my hands on the DigiSpark Attiny85 USB that was exactly what I did. After I was finished I realized that I actually made a fun project that others might enjoy. So that’s what I’m doing. So lets jump into it.
First thing you are going to need is the Attiny85 USB. They are super cheap, mine came in a pack of 5 for around $20. Now installing the drivers can be a pain, but I will walk you through it real fast and point out some common problems. You need to download the drivers from Github. Unzip and install the DPinst64 file. If the Attiny is not recognized you will need to go into your device manager and click View, Show Hidden Devices then with it plugged in look under “Other Devices” and you should see it names DigiSpark. Click on it and select update drivers and select the driver you unzipped. Here is the problem I had. If you do NOT here the connection sound when you plug it in the try a different usb port. I had to use the port on the back of my computer to this, later getting my USB dock that I plugged into the back of my computer.
To do that go in the Arduino IDE and go to File/Preferences and add http://digistump.com/package_digistump_index.json to the Additional boards manager URLs:

Click on Tools / Boards Manager and search Digistump. Install the Digistump AVR Boards. After that is finished go into your ide and select the Board "DigiSpark (Default - 16.5mhz)".

Now that you have the drivers installed and the board selected its time to write some code and upload it to the board. This script has basic features for keyboard output and covers enough that you can move on to other projects and know how to use the keyboard out. We will start with turning the LED light on so we know are script is running.
#include "DigiKeyboard.h"
void setup()
{
pinMode(1, HIGH);
}
When working with the DigiKeyboard SendKey you need to remember SendKeyPress means it will hold the key without releasing while the SendKeyStroke is a press and release. We will be using both in this script and it is important to note there function. The first thing to do is open the search so we can open programs. Do this with.
#include "DigiKeyboard.h"
void setup()
{
pinMode(1, HIGH);
DigiKeyboard.sendKeyPress(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
}
We add a delay so the computer has time to do what we asked. If you didn’t have the delay it would type faster than the computer could handle. Now we are searching for Adjust Volume and using the Tab key to move to the volume slider. This part might need wrote different depending on your computer. Just navigate to the setting you want using Tab and Backspace to move around noting the order you press them and use that in the code. Once you have volume selected use
SendKeyPress(Key_Right_Arrow) to adjust the slider all the way to the right with a delay of (3000).
The 3000 delay means it will hold the right arrow down for that amount of time. So even if the computer volume is all the way down that will be enough time to max it out.
#include "DigiKeyboard.h"
void setup()
{
pinMode(1, HIGH);
DigiKeyboard.sendKeyPress(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("Adjust Volume");
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_ENTER);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyPress(KEY_RIGHT_ARROW);
DigiKeyboard.delay(3000);
}
Now that the volume is up, we will search again this time searching for Chrome “since that’s what is installed on my computer”.
#include "DigiKeyboard.h"
void setup()
{
pinMode(1, HIGH);
DigiKeyboard.sendKeyPress(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("Adjust Volume");
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_ENTER);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyPress(KEY_RIGHT_ARROW);
DigiKeyboard.delay(3000);
DigiKeyboard.sendKeyPress(0);
DigiKeyboard.sendKeyStroke(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("chrome");
DigiKeyboard.delay(1500);
}
Last step is to paste the link you want to load. In my case I used the classic Rick Astley.
#include "DigiKeyboard.h"
void setup()
{
pinMode(1, HIGH);
DigiKeyboard.sendKeyPress(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("Adjust Volume");
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_ENTER);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.sendKeyStroke(KEY_TAB);
DigiKeyboard.delay(1000);
DigiKeyboard.sendKeyPress(KEY_RIGHT_ARROW);
DigiKeyboard.delay(3000);
DigiKeyboard.sendKeyPress(0);
DigiKeyboard.sendKeyStroke(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("chrome");
DigiKeyboard.delay(1500);
DigiKeyboard.println("https://www.youtube.com/watch?v=dQw4w9WgXcQ&ab_channel=RickAstley");
}
That wraps it up. Pretty simple and easy to modify. You could move the search for chrome and the site link into the loop function with a delay function to open windows over and over.
Add a list of links and randomly pick 1 from a list to give someone a really hard time. This project is really just to have fun and get you used to the Attiny85 features. I hope you enjoy it!
void loop()
{
DigiKeyboard.sendKeyStroke(KEY_S, KEY_LEFT_GUI);
DigiKeyboard.delay(1000);
DigiKeyboard.println("chrome");
DigiKeyboard.delay(1500);
DigiKeyboard.println("https://www.youtube.com/watch?v=dQw4w9WgXcQ&ab_channel=RickAstley");
delay(5);
}